HTML Buttons - A Developer's Guide
Buttons are everywhere on the web - whether you're submitting a form, triggering a script, or navigating to another page, buttons play a crucial role in user interaction.
Despite their simple appearance, HTML buttons offer a lot of versatility and complexity. You can design buttons using an actual button element or a variety of other html elements depending on the use case.
Button design can also have a huge impact on how your users engage with your website and valuable considerations around things like conversion rate.
In this guide, we explore how buttons work and how you can code them from a technical perspective and then we'll get hands on with some cool CSS button styles looking at examples and how you can easily style buttons too.
If you're a seasoned coder, the guide gets more heavy duty further in and anyone can jump into the button generator to whip up HTML buttons in moments.
What are HTML Buttons?
At their core, HTML buttons are interactive elements that allow users to engage with a web page.
Whether they're used to submit a form, activate javascript code, or link to another page, buttons provide a clear call to action. These buttons can be created using several different HTML elements, with the <button>
tag being the best semantic match but the anchor tag <a>
is commonly used as well as the input element with the type attribute set to 'submit'.
While they might look simple on the surface, understanding how to use buttons effectively can improve both the functionality and design of your site.
How to code a HTML button element
A very simple button element might look like this:
<button type="button">Click Me!</button>
Don't forget the opening and closing tags when using the button element. Opening tag: <button>
, ending tag: </button>
.
Initiating a Javascript code snippet with the button element
This button element coded as it is, won't actually do anything. We need to add some functionality to it. The button element is perfect for initiating javascript code on your webpage such as:
<button type="button" onclick="alert('Button clicked!')">Click Me!<button>
With the button onclick attribute used, the button element becomes a clickable button that performs a function. In this case it 'alerts' the user and provides the message in that alert box 'Button Clicked'.
You can also move the javascript code out of the button and into a separate script tag for more complex scripting as in this code snippet:
<button type="button" onclick="button_click()">Click Me!</button>
<script>
function button_click() {
alert('The function ran when the button was clicked');
}
</script>
Other uses for the HTML Button tag <button> with Javascript
The button element can be put to a multitude of uses with javascript. Trigger a popup, submit a form or use it to apply styles to your page changing the appearance. Button elements also have other functions for handling form data which we'll cover later on.
Here are example code snippets for how to trigger a popup, submit a form or apply styles.
Trigger Popup
<button type="button" onclick="trigger_popup()">Open Modal</button>
<id="popup" style="display:none;">
<!-- Modal html content here -->
</div>
<script>
function trigger_popup() {
document.getElementById('popup').style.display = 'block';
}
</script>
In this example, the button onclick initiates the function 'trigger_popup' which simply gives the element with the ID 'popup' the inline style of 'block' which overwrites the initial value of display 'none'.
Submit form data
Inside a HTML form <form> we can use a button tag <button> to submit the form and all the form data in one of two ways. Either with a javascript function or by defining a button type 'submit'.
Both of these methods will result in the user submitting the form but you can add additional functions to the Javascript code if you are using that method whereas with the 'type="submit"' method you are limited to simply submitting the form come what may.
Button type attribute 'submit'
Here's an example form with a submit button tag and the type attribute set to 'submit':
<form action="/submit" method="post">
<input type="text" name="name" placeholder="Enter your name"/>
<button type="submit">Submit Form</button>
</form>
You can use the <button> element interchangeably with an <input type="submit" value="Submit Form"> for form submissions. The advantage of using <button type="submit"> is that you can include more complex content, such as images, icons, or additional HTML, inside the button.
The HTML Buttons framework, available on this website, works in forms with both the <button> and the <input type="submit"> element like this example to make a useful submit button.
<form action="/submit" method="post">
<input type="text" name="name" placeholder="Enter your name"/>
<div class="html_button btn-left">
<button type="submit" class="btn my_button large">Submit Form</button>
<!-- OR... -->
<input type="submit" class="btn my_button large" value="Submit Form"/>
</div>
</form>
Whether you use the button tag or the input element for your submit button, when using the HTML Buttons CSS framework, they will both look exactly the same on the page. Note the input element is a self closing tag.
Buttons can also be configured to reset the user's form data like this example:
<div class="html_button btn-left">
<button type="reset" class="btn my_button large">Reset Form</button>
</div>
How to style a HTML button tag with CSS
Making html elements look good is a bit of an art form but one we will hopefully make easier for anyone, artist or not, to do today.
Once we have our html elements in place, we want to to turn them into buttons, as we imagine them to look, with CSS.
There are two types of HTML button really. One is the actual html <button> tag that is the proper definition of the element and then there's anything that we want to make look like a button using any HTML elements that fit the use case.
HTML Buttons offers a framework for designing buttons that have a consistent appearance no matter what element you need to use. The button generator makes is quick and easy to style buttons for your project and to copy the code snippet.
There are lots of factors to consider when styling a button such as background color how you align items, font size, font family, padding, margin and more.
Background Color
The background color of your button usually needs to stand out. As UX designers we often really want users to click on a specific button as a call to action and for that to happen the button needs to be prominent.
So, the background color typically wants to contrast well with whatever it's in or on. If the button sits over a very dark image then make the background color bright. If the button sits over a light element, make the background color of the button tag darker.
However, the background color also needs to contrast sufficiently with the text inside the button tag so it is readable.
Here are some example buttons styled using the HTML Button generator with good background color contrast.
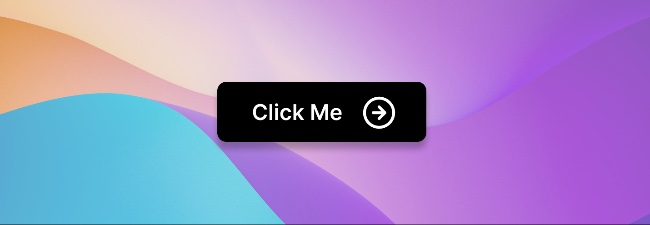
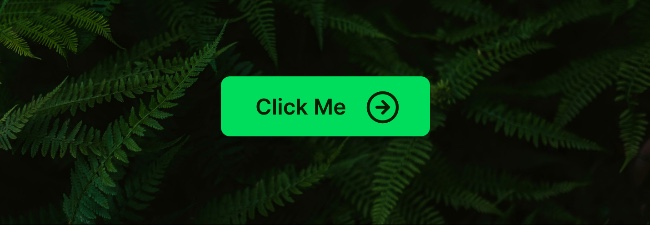
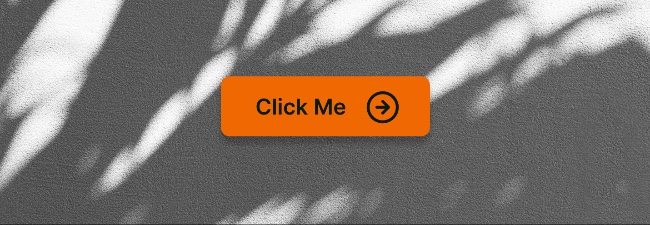
Writing CSS code for background color
To style the background color of your button, you can add the background-color property:
<style>
button {
background-color:red;
}
</style>
When using the HTML buttons framework, the background-color property is defined alongside other properties inside a specific class for the button you have designed:
<style>
.my_new_button {
background-color:blue;
/*-- other properties. --*/
}
</style>
The background-color property is universally supported across all modern web browsers, including Chrome, Firefox, Safari, Edge, and even older versions like Internet Explorer (from version 4 onwards). It’s one of the core CSS properties that has been consistently supported for a long time.
Styling Text for your button
Getting the style of your html content or inner button text right has a big impact on the way your button tag looks. The text align, text decoration, font size and font family can all be defined. Usually, the inner html elements of your button tag are inline block but they don't have to be.
Text Align
The text-align property in CSS is used to specify how inline content (like text) is aligned within a block element (like your button). If your button's background wraps around the text, changing the text alignment inside the button might not achieve anything. If your button is filling all the available space then changing the text alignment inside the button tag would have a noticeable effect.
When using the HTML Buttons framework, the
<div class="html_button btn-fill">
<button type="button" onclick="func()" class="btn my_button large">Click Me</button>
</div>
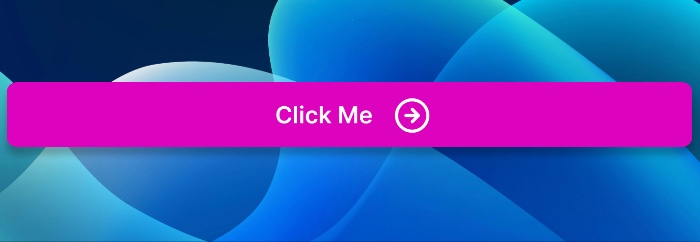
The CSS here has the text align set to centre but you can align items right, left, centre or justify.
.my_button {
text-align: center;
/*-- text-align: left | right | justify; --*/
}
Text Decoration
The text-decoration property has been expanded in CSS3 to include more detailed customization using sub-properties. You can now specify the color of the decoration (underline, overline, line-through), independent of the text color which is cool.
.my_button {
text-decoration: underline;
text-decoration-style: wavy;
text-decoration-color: green;
}
Font size
Choosing the right font size for your button tag is relative to the rest of your design, but, in a similar way to the background color if you want the button to be seen and clicked on, the font size needs to stand out.
If your button needs to be a more subtle piece of your UX then the font size can be more inline with other elements around it. In any case, here's how to adjust the font size of your button using CSS:
.my_button {
font-size:20px;
}
Font Family
Your design overall will likely have a font family already defined and that's great, you can use that font family in your button like any other html elements.
However, if your chosen font is hard to read, especially on mobile, and you want a very clickable button then use a font-family that is easy to read. This typically means the font is sans-serif but it doesn't have to.
Here is an article that gives loads of example button designs that use serif and display fonts really well.
The HTML Button generator let's you try out different fonts while you are designing your button to see which ones work best. The generator loads up fonts from Google Fonts so they are easy to use in your project overall. For more help on using custom fonts in your project, check out our 'installing Google Fonts with HTML Buttons on your website' article.
Here's how to adjust the font family of your button tag with CSS:
.my_button {
font-family: 'Open Sans', sans-serif;
}
Border Radius
Too much border radius or too little? It's tricky to get right. The HTML Button Generator lets you adjust the border radius with a slider so you can adjust it until its just right.
A lot of buttons you see online have rounded corners. Something about rounding the corners signals that this object or box is a clickable button. Here's how to add border radius to your button tag using CSS:
.my_button {
border-radius:10px;
}
Box Shadow
Box shadow lifts a button off the page and gives it the appearance of floating or hovering. Box shadow is a subtle but powerful signal that the element is a clickable button in UX design.
Box shadow is made up of horizontal offset, vertical offset, blur radius, spread radius and color. These properties combine to create what looks like a real-life shadow cast by your floating button. The need to press the button becomes quite irresistible!
Luckily the HTML Button Generator can configure box shadow really easily and add all the right properties to your button's class.
.my_button {
box-shadow: 2px 4px 8px rgba(0, 0, 0, 0.3);
}
Pseudo CSS Classes for the button element
Buttons are interactive elements, so they naturally respond to user actions like hovering, clicking, and focusing. CSS pseudo-classes help you style these states, providing important visual feedback to the user. There are three main pseudo-classes to be aware of when styling buttons:
Hover: When the user moves their cursor over the button, it triggers the hover state. This is often used to subtly change the button’s appearance and signal that it's clickable.
.my_button:hover {
background-color: darkblue;
}
Active: The active state occurs when the button is being pressed (while the mouse button is held down). It’s common to give the button a pressed effect, such as changing the color or reducing its shadow.
.my_button:active {
background-color: navy;
}
Focus: The focus state is triggered when a button gains focus, either by clicking it or navigating to it via the keyboard (usually with the Tab key). Adding a visible outline or border for focus helps with accessibility, especially for keyboard navigation.
.my_button:focus {
outline: 2px solid orange;
}
Using these pseudo-classes improves the overall user experience by making your buttons feel more interactive and accessible. The HTML button generator lets you configure these pseudo states visually and outputs the necessary CSS.
CSS Transitions
Adding CSS transitions to your buttons can enhance the user experience by making state changes (like hover or active) feel smooth and natural. Instead of snapping instantly between styles, transitions allow the button to gradually change over a set time. This subtle effect can make your styled button feel more polished and responsive.
You can apply transitions to many different CSS properties, such as background-color, box-shadow, border-radius, and more. Here’s how you can use a transition for the background-color and box-shadow properties:
.my_button {
background-color: blue;
box-shadow: 2px 4px 8px rgba(0, 0, 0, 0.3);
transition: background-color 0.3s ease, box-shadow 0.3s ease;
}
.my_button:hover {
background-color: darkblue;
box-shadow: 4px 8px 16px rgba(0, 0, 0, 0.4);
}
In this example:
- transition: background-color 0.3s ease, box-shadow 0.3s ease; smoothly animates both the background-color and box-shadow changes over 0.3 seconds.
- The ease timing function starts slow, speeds up in the middle, and slows down again, creating a smooth transition.
Transitions can make even simple style changes, like color or shadow effects, more visually appealing and engaging for users.
The HTML button generator lets you configure these transition properties visually and outputs the necessary CSS style so you can add your styled button to the website.
Using the HTML Buttons Generator
The HTML Button generator can be used to configure the appearance and behaviour of buttons easily and quickly. As you choose style and function options for your button, the generator shows you what your button will look like, as you design it.
Once you're happy with your button design, you can copy the HTML and CSS to add to your website.
The HTML Buttons framework takes care of lots of the detail required for making good buttons. It's a very user-friendly way to code buttons for your website whether you're a seasoned pro or just starting out.
The generator takes care of accessibility and cross browser support too.
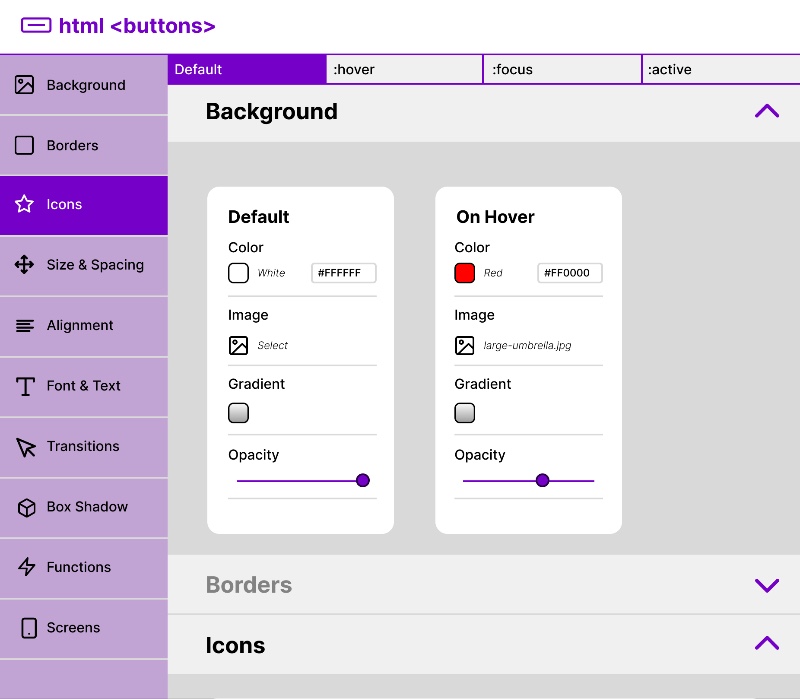
Anchor tag versus button tag
When you just want to navigate your user to a different page, the anchor tag is always the most efficient and semantically correct tag to use. Your button can still look like a button without actually having to be a button tag.
Anchor and button tags usually have different default styling in browsers. Anchor tags tend to have their display property set to 'inline' where as the button tag tends to have it set to 'block' or 'inline block'.
To use the anchor tag as a button that stands out from other html elements on your page, you'll probably need to adjust the display property. The HTML Buttons framework handles this for you.
Here's what a button as a anchor tag looks like in the code snippet:
<div class="html_button btn-left">
<a href="#" class="btn my_button large">Click me</a>
</div>
HTML Button States and Attributes
Buttons in HTML can have different states that affect how they behave or how they are styled. These states provide important functionality and are controlled by specific attributes. In this section, we’ll cover three key attributes disabled and autofocus and explain how they work with code examples.
Disabled Attribute
The disabled attribute is used to make a button non-clickable or no longer an interactive element. When a button is disabled, it won’t respond to user interactions, and browsers typically apply a default "grayed out" style to indicate its inactive state.
When you add the disabled attribute to a button, it becomes unclickable and cannot be focused or activated by any means. This is useful when certain actions should be unavailable until a condition is met (e.g., completing a form).
When a button is disabled, it typically appears grayed out and is non-clickable by default. You can further style disabled buttons using the :disabled pseudo-class.
<div class="html_button btn-left">
<button type="button" class="btn my_button large" disabled>Click me</button>
</div>
.my_button:disabled {
background-color: #cccccc; /* Gray background */
color: #666666; /* Gray text */
cursor: not-allowed; /* Show 'not-allowed' cursor */
opacity: 0.5; /* Slightly transparent */
border: 1px solid #999999; /* Lighter border */
}
Autofocus Attribute
The autofocus attribute automatically focuses the button (or any other focusable element) when the page loads. This is helpful in cases where you want a particular button to be pre-selected or highlighted for the user’s convenience.
Adding autofocus to a button causes the browser to focus on that button as soon as the page loads.
Only one element on the page can have the autofocus attribute, and if more than one element has it, the browser will focus on the first one.
<div class="html_button btn-left">
<button type="button" class="btn my_button large" autofocus>Click me</button>
</div>
.my_button:focus {
outline: 3px solid red; /* Add a bright orange outline */
background-color: yellow; /* Highlight background on focus */
}
The autofocus attribute doesn't have a specific pseudo-class, but you can target it using the :focus pseudo-class as in the above example. This allows you to style how the button looks when it automatically gains focus on page load.
The HTML Button Generator outputs the necessary CSS for the disabled and autofocus attribute if you need it and helps make it easy to visualise what your button will look like in different states.
Buttons and Accessibility
When designing websites, it’s essential to ensure that your HTML buttons are accessible to all users, including those who rely on assistive technologies such as screen readers, keyboard navigation, or voice commands.
Accessible buttons improve user experience by ensuring that they are operable and understandable for everyone, regardless of their physical or cognitive abilities. In this section, we’ll cover key practices for making your buttons more accessible.
Semantic HTML elements
Always use the proper HTML element for buttons. The <button> tag is naturally recognized by assistive technologies and provides built-in accessibility features. Avoid using non-semantic elements like
If you use a non-semantic element (like an <a> tag), you must ensure the screen reader understands it as a button:
<div class="html_button btn-left">
<a href="#" role="button" aria-label="Click to submit">Submit</a>
</div>
Use the HTML Button Generator to generate an accessible button element whether it's an anchor tag or a button tag or an input type button.
Clear Labelling
Your button tag will need clear, concise, and descriptive labels so that screen readers can announce their purpose. This is typically done by the button’s text content, but you can also use aria-label or aria-labelledby for additional context.
- aria-label: Provides a custom label for screen readers.
- aria-labelledby: Links the button to another element, like a heading, for more detailed labeling.
Ensure Buttons Are Keyboard Accessible
Users who rely on keyboard navigation must be able to interact with buttons using the Tab key to focus and the Enter or Spacebar keys to activate them. By default, <button> elements are keyboard accessible. However, you should ensure that any custom buttons or interactive elements that look like buttons (e.g., styled <a> tags or <div>s) are also focusable and operable via the keyboard.
To make a non-semantic element accessible via keyboard, you need to set:
- tabindex="0": This makes the element focusable with the keyboard.
- role="button": This tells assistive technologies that the element should be treated as a button.
<div class="html_button btn-left">
<div role="button" tabindex="0" aria-label="Custom button">Click Me</div>
</div>
Provide Sufficient Color Contrast for accessibility purposes
Ensure your buttons have sufficient color contrast between their background and text. This is particularly important for users with low vision or color blindness. The Web Content Accessibility Guidelines (WCAG) recommend a contrast ratio of at least 4.5:1 for normal text.
The HTML Button generator checks button designs and lets you know if the button you are designing has enough contrast and meets accessibility standards.
Text Size and Clickable Area
Ensure that button text is large enough to be easily readable, and that the button’s clickable area is large enough for users with motor impairments to interact with. WCAG recommends that clickable areas should be at least 44x44 pixels in size.
Provide Feedback for Button Actions
Buttons that perform actions should provide feedback to the user, such as changing appearance on click (using pseudo-classes like :active) or showing a success message after submission. This visual feedback reassures users that their interaction was successful.
The HTML Button generator can help you create hover, active and focus states for your button element easily.
Accessibility and Cross-browser Consistency
For accessibility, HTML buttons are well-supported with assistive technologies across major browsers, provided that:
- The <button> element is used semantically for actual buttons.
- Buttons are given clear labels, either with text content or an accessible label (e.g., using aria-label).
Additionally, modern browsers handle accessibility features such as aria attributes and proper focus management well. Always ensure buttons can be operated by keyboard alone (focus states), and test in multiple browsers and screen readers to ensure consistent accessibility support.
The type attribute on a button (e.g., type="submit", type="button", or type="reset") is primarily used to define the button's behavior in forms, not to help a screen reader identify the element.
Screen readers rely more on the HTML element itself (such as the <button> tag) and other accessibility attributes like aria-label, role, and aria-describedby to provide useful information to users with disabilities.
Buttons and Page Performance
The HTML Buttons generator and framework aims to deliver buttons that are very lightweight, fast to load and do not limit your page performance in any way.
We do this by using nothing but native HTML and CSS and never any images to render buttons. This reduces network requests and downloading images. HTML Buttons render as part of the normal document cascade.
We also embed any icons into the CSS so you don't need external icon libraries that significantly impact page performance by adding in unnecessary network requests.
We also use native CSS transitions and no javascript-heavy animations for the button's effects.
Designing and styling buttons using just HTML, CSS, and JavaScript provides significant performance advantages over other methods.
The HTML Button Generator reduces page load times, minimises external resource dependencies, and ensures that your buttons are responsive and fast on all devices. By choosing to style buttons without unnecessary libraries or assets, you can enhance your website's overall performance while maintaining a clean and professional design.
Buttons and Browser support
When building buttons for your website, it's essential to understand how HTML buttons behave across different browsers and platforms. HTML buttons are widely supported, but there are a few nuances to keep in mind to ensure consistent behavior across all users' devices.
Basic Browser Support for HTML Buttons
HTML buttons, such as the <button> and <input> element, are universally supported across all modern browsers, including:
- Google Chrome
- Mozilla Firefox
- Safari (both macOS and iOS)
- Microsoft Edge
- Opera
Even older browsers, such as Internet Explorer 11, have browser support basic button elements, making them reliable for nearly any web project. The core button type attribute and functionality, such as type="submit", type="button", and even JavaScript event listeners like onclick, work consistently across these browsers.
Mobile Browser Support
Mobile browsers, including Chrome for Android, Safari on iOS, and other mobile browsers like Firefox for Android, also fully support HTML buttons. Buttons typically behave as expected on touch interfaces, responding to taps instead of clicks. If using hover effects, keep in mind that on mobile devices, hover states are usually triggered after tapping and held until the next action, as there is no mouse cursor to "hover" over elements.
CSS Styling and Browser Differences
When it comes to styling buttons with CSS, browsers generally support the following properties without issue:
- background-color
- border
- font-size
- box-shadow
- text-align
- border-radius
However, there may be minor rendering differences between browsers when it comes to the default appearance of buttons, especially the <input> and <button> elements. For example, buttons in Safari and iOS may have a slightly different default padding and border style than those in Chrome or Firefox. To avoid inconsistencies, it's a good practice to normalize button styles with CSS.